Abstract: Without a dedicated bus master (such as DS2480B, DS2490), the microprocessor can easily generate 1-Wire timing signals. This application note gives an example of a basic subroutine for 1-Wire master communication written in 'C' that supports standard rates. The four basic operations of the 1-Wire bus are: reset, write "1", write "0", and read data bits. Byte operation can be achieved by repeatedly calling bit operations. This article provides the time parameters for reliable communication with 1-Wire devices through various transmission lines.
Introduction In the absence of a dedicated bus master, the microprocessor can easily generate 1-Wire timing signals. This application note gives an example of a basic subroutine for 1-Wire master communication written in 'C' that supports standard rates. In addition, this article also discusses the high-speed communication mode. For the code in this example to function properly, the system must meet the following requirements:
The communication port of the microprocessor must be bidirectional, its output is open drain, and there is a weak pull-up on the line. This is also a basic requirement for all 1-Wire buses. For a simple example of a 1-Wire host microprocessor circuit, see Appendix A of the 1-Wire Network Reliable Design Guide (Application Note 148). The microprocessor must be able to generate the precise 1µs delay required for standard speed 1-Wire communication and the 0.25µs delay required for high speed communication. The communication process cannot be interrupted. The 1-Wire bus has four basic operations: reset, write 1 bit, write 0 bit, and read bit operation. In the product data, the time to complete a bit transmission is called a time slot. So byte transfer can be achieved by calling bit operations multiple times. Table 1 below is a brief description of each bit operation and a list of steps necessary to implement these operations. Figure 1 is its timing waveform diagram. Table 2 gives the shortest, longest and recommended time for the 1-Wire master to communicate with the 1-Wire device under normal line conditions. If the device connected to the 1-Wire master is special or the line conditions are special, the minimum or maximum value can be used.
Table 1. 1-Wire operation
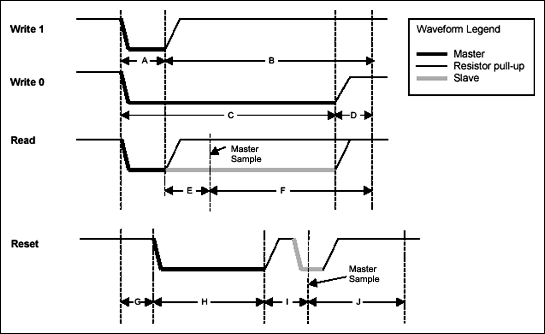
Figure 1. 1-Wire timing diagram
Table 2. 1-Wire master timing
For detailed calculation of these values ​​in the table, please refer to: http://files.dalsemi.com/auto_id/public/an126.zip
Note: In the product data sheet, it is expressed as tW1L (write 1 low) minus ε (time to rise to VTH) plus tF (time to fall to VTL). The network is assumed to be a medium-distance network of standard speed, and the rise and fall times do not exceed 3 µs. Assume that the network is a small, high-speed network with rise and fall times not exceeding 0.5µs. In the data, it is expressed as tSLOT (slot time) minus the time represented by 'A'. In the data, it is expressed as tW0L (write 0 low) minus δ (time to rise to VIHMASTER) plus tF (time to fall to VTL). In the data, it is expressed as tREC (recovery time) plus δ (time to rise to VIHMASTER). In the data, it is expressed as tMSR (host sample read time) plus tF (time to fall to VTL), and then subtract 'A'. Within this range, sampling should be as late as possible in order to obtain the longest recovery time. In the data, it is expressed as tSLOT (slot time) minus 'A', and then minus 'E'. In the data sheet, it is expressed as tRSTL (time to reset to low) minus ε (time to rise to VTH) plus tF (time to fall to VTL). In the data, it is expressed as tMSP (master sample response time) plus ε (time to rise to VTH). In the data, the minimum value is expressed as tRSTL (reset low time) minus the time taken by 'I'. The 1-Wire reset operation mentioned here does not take into account the extended acknowledgment (alarm) pulse sequence used by DS2404 and DS1994. For this special case, please refer to the product data sheet. Sampling at the end of the 1-Wire reset sequence can verify that the 1-Wire bus has returned to the pull-up level. If the level is still 0, it may be a short circuit between the 1-Wire bus and ground, or the DS2404 / DS1994 issued an alarm signal. Expressed as tREC (Recovery Time) minus the time taken for 'D'. In high-speed applications, some devices require additional delay before tRSTL (low-level reset time) to ensure that the parasitic power supply of the device is fully charged. For low voltage operation, some devices may require a longer delay. Refer to the device data sheet for appropriate parameter values. Code examples The following code examples rely on two general 'C' functions outp and inp to read and write byte data from the IO port. They are usually located In the standard library. When applied to other platforms, you can use appropriate functions to replace them.
// send 'databyte' to 'port' int outp (unsigned port, int databyte); // read byte from 'port' int inp (unsigned port); the constant PORTADDRESS (Figure 3) in the code is used to define the communication port address. Here we assume that bit 0 of the communication port is used to control the 1-Wire bus. Setting this bit to 1 will cause the 1-Wire bus to go low; setting this bit to 0 will release the 1-Wire bus, and the 1-Wire bus will be pulled up by the resistor or 1-Wire Pull down from the device.
The tickDelay function in the code is a user-programmed subroutine. This function is used to generate a delay of an integer multiple of 1 / 4µs. Under different platforms, the implementation of this function is also different, so it will not be described in detail here. The following is the tickDelay function declaration code, and a SetSpeed ​​function, which is used to set the delay time for standard speed and high-speed mode.
Example 1. 1-Wire timing generation // Pause for exactly 'tick' number of ticks = 0.25us void tickDelay (int tick); // Implementation is platform specific // 'tick' values ​​int A, B, C, D , E, F, G, H, I, J; // ------------------------------------ ------------------------------------------ // Set the 1-Wire timing to 'standard' (standard = 1) or 'overdrive' (standard = 0). // void SetSpeed ​​(int standard) {// Adjust tick values ​​depending on speed if (standard) {// Standard Speed ​​A = 6 * 4 ; B = 64 * 4; C = 60 * 4; D = 10 * 4; E = 9 * 4; F = 55 * 4; G = 0; H = 480 * 4; I = 70 * 4; J = 410 * 4;} else {// Overdrive Speed ​​A = 1.5 * 4; B = 7.5 * 4; C = 7.5 * 4; D = 2.5 * 4; E = 0.75 * 4; F = 7 * 4; G = 2.5 * 4; H = 70 * 4; I = 8.5 * 4; J = 40 * 4;}} The code program for basic 1-Wire operation is shown in Example 2.
Example 2. Basic 1-Wire functions // -------------------------------------------------- -------------------------------------- // Generate a 1-Wire reset, return 1 if no presence detect was found, // return 0 otherwise. // (NOTE: Does not handle alarm presence from DS2404 / DS1994) // int OWTouchReset (void) {int result; tickDelay (G); outp (PORTADDRESS, 0x00); // Drives DQ low tickDelay (H); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (I); result = inp (PORTADDRESS) & 0x01; // Sample for presence pulse from slave tickDelay (J); / / Complete the reset sequence recovery return result; // Return sample presence pulse result} // ------------------------------- ---------------------------------------------- // Send a 1-Wire write bit. Provide 10us recovery time. // void OWWriteBit (int bit) {if (bit) {// Write '1' bit outp (PORTADDRESS, 0x00); // Drives DQ low tickDelay (A); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (B); // Complete the time slot and 10us recovery} else {// Write '0' bit outp (POR TADDRESS, 0x00); // Drives DQ low tickDelay (C); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (D);}} // -------------- -------------------------------------------------- ------------- // Read a bit from the 1-Wire bus and return it. Provide 10us recovery time. // int OWReadBit (void) {int result; outp (PORTADDRESS, 0x00) ; // Drives DQ low tickDelay (A); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (E); result = inp (PORTADDRESS) & 0x01; // Sample the bit value from the slave tickDelay (F) ; // Complete the time slot and 10us recovery return result;} This program includes all bit operations on the 1-Wire bus. By calling this program, you can form a function that takes bytes as the processing object, see Example 3.
Example 3. Derived 1-Wire function // ------------------------------------------ -------------------------------------- // Write 1-Wire data byte // void OWWriteByte (int data) {int loop; // Loop to write each bit in the byte, LS-bit first for (loop = 0; loop <8; loop ++) {OWWriteBit (data & 0x01); // shift the data byte for the next bit data >> = 1;}} // ------------------------------------- ---------------------------------------- // Read 1-Wire data byte and return it // int OWReadByte (void) {int loop, result = 0; for (loop = 0; loop <8; loop ++) {// shift the result to get it ready for the next bit result >> = 1; // if result is one, then set MS bit if (OWReadBit ()) result | = 0x80;} return result;} // ----------------------- -------------------------------------------------- ---- // Write a 1-Wire data byte and return the sampled result. // int OWTouchByte (int data) {int loop, result = 0; for (loop = 0; loop <8; loop ++) {// shift the result to get it ready for the next bit result >> = 1; // If sending a '1' then read a bit else write a '0' if (data & 0x01) {if (OWReadBit ()) result | = 0x80;} else OWWriteBit (0); // shift the data byte for the next bit data >> = 1;} return result;} // ------------------------------------- ---------------------------------------- // Write a block 1-Wire data bytes and return the sampled result in the same // buffer. // void OWBlock (unsigned char * data, int data_len) {int loop; for (loop = 0; loop <data_len; loop ++) {data [loop] = OWTouchByte (data [loop]);}} // ----------------------------------------- ------------------------------------ // Set all devices on 1-Wire to overdrive speed. Return '1' if at least one // overdrive capable device is detected. // int OWOverdriveSkip (unsigned char * data, int data_len) {// set the speed to 'standard' SetSpeed ​​(1); // reset all devices if ( OWTouchReset ()) // Reset the 1-Wire bus return 0; // Return if no devices found // overdrive skip command OWWriteByte (0x3C); // set the speed to 'overdrive' SetSpeed ​​(0 ); // do a 1-Wire reset in 'overdrive' and return presence result return OWTouchReset ();} The OWTouchByte function can finish reading and writing 1-Wire bus data at the same time, and can read and write data blocks through this function. The execution efficiency is higher on some platforms, and the API provided by Maxim uses this function. Through the OWTouchByte function, the OWBlock function simplifies the sending and receiving of data blocks on the 1-Wire bus. Note: OWTouchByte (0xFF) is equivalent to OWReadByte (), and OWTouchByte (data) is equivalent to OOWriteByte (data).
These functions and the tickDelay function together form all functions necessary for 1-Wire bus to perform bit, byte and block operations. Example 4 shows an example of using these functions to read the DS2432's SHA-1 authentication page.
Example 4. Read the DS2432 example // ------------------------------------- ----------------------------------- // Read and return the page data and SHA-1 message authentication code (MAC) // from a DS2432. // int ReadPageMAC (int page, unsigned char * page_data, unsigned char * mac) {int i; unsigned short data_crc16, mac_crc16; // set the speed to 'standard' SetSpeed ​​(1) ; // select the device if (OWTouchReset ()) // Reset the 1-Wire bus return 0; // Return if no devices found OWWriteByte (0xCC); // Send Skip ROM command to select single device // read the page OWWriteByte (0xA5); // Read Authentication command OWWriteByte ((page << 5) & 0xFF); // TA1 OWWriteByte (0); // TA2 (always zero for DS2432) // read the page data for (i = 0 ; i <32; i ++) page_data [i] = OWReadByte (); OWWriteByte (0xFF); // read the CRC16 of command, address, and data data_crc16 = OWReadByte (); data_crc16 ; // delay 2ms for the device MAC computation // read the MAC for (i = 0; i <20; i ++) mac [i] = OWReadBy te (); // read CRC16 of the MAC mac_crc16 = OWReadByte (); mac_crc16 | = (OWReadByte () << 8); // check CRC16 ... return 1;} additional softwareThis application note gives 1- The basic functions of the Wire bus operation. These basic functions are the basis for building complex 1-Wire applications. An important operation ignored in this article is 1-Wire search. Through 1-Wire search, you can search for the unique ID numbers of multiple 1-Wire slave devices attached to the bus. This search method is described in detail in Application Note 187: "1-Wire Search Algorithm", and C code that implements these 1-Wire basic functions is also given.
The 1-Wire public development kit contains a lot of code written for specific devices, provided by the following links:
http: //
For more detailed information, please refer to Application Note 155: "1-Wire Software Resource Guide".
Alternative solution If it is not feasible to implement the 1-Wire master solution through software for a specific application, then as an alternative solution, a 1-Wire master chip or a synthesized 1-Wire master unit can be used.
Maxim provides 1-Wire masters written in Verilog and VHDL.
DS1WM
To obtain the Verilog / VHDL code of the 1-Wire host, please submit a technical support application.
The working method of the synthesized 1-Wire master is described in application note 120: "Communication with 1-Wire Master" and application note 119: "Embedded 1-Wire Master".
There are a variety of 1-Wire host chips that can be used as peripherals for microprocessors. The serial, 1-Wire line driver DS2480B can be easily connected to a standard serial port.
For details on the operation of the DS2480B, see Application Note 192: "Use of the DS2480B Serial Interface 1-Wire Line Driver").
This is an advanced 1-Wire line driver specially designed for long-distance 1-Wire transmission lines. In application note 244: "Excellent 1-Wire Network Driver", a more detailed 1-Wire design specifically designed for long lines is described. Wire drive.
Revision history
07/06/00: Version 1.0-the original version.
05/28/02: Version 2.0-Corrected 1-Wire reset sampling time. Added waveform diagrams, links, and more code examples.
02/02/04: Version 2.1-Added support for high-speed mode, given minimum and maximum timings, and updated code examples.
09/06/05: Version 2.2-Corrected PIO polarity in the code routine description.
Introduction In the absence of a dedicated bus master, the microprocessor can easily generate 1-Wire timing signals. This application note gives an example of a basic subroutine for 1-Wire master communication written in 'C' that supports standard rates. In addition, this article also discusses the high-speed communication mode. For the code in this example to function properly, the system must meet the following requirements:
The communication port of the microprocessor must be bidirectional, its output is open drain, and there is a weak pull-up on the line. This is also a basic requirement for all 1-Wire buses. For a simple example of a 1-Wire host microprocessor circuit, see Appendix A of the 1-Wire Network Reliable Design Guide (Application Note 148). The microprocessor must be able to generate the precise 1µs delay required for standard speed 1-Wire communication and the 0.25µs delay required for high speed communication. The communication process cannot be interrupted. The 1-Wire bus has four basic operations: reset, write 1 bit, write 0 bit, and read bit operation. In the product data, the time to complete a bit transmission is called a time slot. So byte transfer can be achieved by calling bit operations multiple times. Table 1 below is a brief description of each bit operation and a list of steps necessary to implement these operations. Figure 1 is its timing waveform diagram. Table 2 gives the shortest, longest and recommended time for the 1-Wire master to communicate with the 1-Wire device under normal line conditions. If the device connected to the 1-Wire master is special or the line conditions are special, the minimum or maximum value can be used.
Table 1. 1-Wire operation
OperaTIon | DescripTIon | ImplementaTIon |
Write 1 bit | Send a '1' bit to the 1-Wire slaves (Write 1 TIme slot) | Drive bus low, delay A Release bus, delay B |
Write 0 bit | send a '0' bit to the 1-Wire slaves (Write 0 time slot) | Drive bus low, delay C Release bus, delay D |
Read bit | Read a bit from the 1-Wire slaves (Read time slot) | Drive bus low, delay A Release bus, delay E Sample bus to read bit from slave Delay F |
Reset | Reset the 1-Wire bus slave devices and ready them for a command | Delay G Drive bus low, delay H Release bus, delay I Sample bus, 0 = device (s) present, 1 = no device present Delay J |
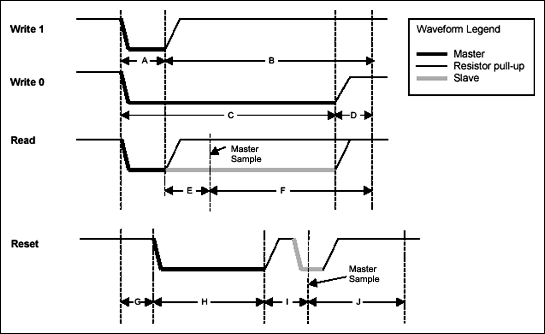
Figure 1. 1-Wire timing diagram
Table 2. 1-Wire master timing
Parameter | Speed | Min (µs) | Recommended (µs) | Max (µs) | Notes |
A | Standard | 5 | 6 | 15 | 1, 2 |
Overdrive | 1 | 1.5 | 1.85 | 1, 3 | |
B | Standard | 59 | 64 | N / A | twenty four |
Overdrive | 7.5 | 7.5 | N / A | 3, 4 | |
C | Standard | 60 | 60 | 120 | 2, 5 |
Overdrive | 7 | 7.5 | 14 | 3, 5 | |
D | Standard | 8 | 10 | N / A | 2, 6 |
Overdrive | 2.5 | 2.5 | N / A | 3, 6 | |
E | Standard | 5 | 9 | 12 | 2, 7, 8 |
Overdrive | 0.5 | 0.75 | 0.85 | 3, 7, 8 | |
F | Standard | 50 | 55 | N / A | 2, 9 |
Overdrive | 6.75 | 7 | N / A | 3, 9 | |
G | Standard | 0 | 0 | 0 | |
Overdrive | 2.5 | 2.5 | N / A | 3, 14 | |
H | Standard | 480 | 480 | 640 | 2, 10, 15 |
Overdrive | 68 | 70 | 80 | 3, 10 | |
I | Standard | 63 | 70 | 78 | 2, 11 |
Overdrive | 7.2 | 8.5 | 8.8 | 3, 11 | |
J | Standard | 410 | 410 | N / A | 2, 12, 13 |
Overdrive | 39.5 | 40 | N / A | 3, 12 |
For detailed calculation of these values ​​in the table, please refer to: http://files.dalsemi.com/auto_id/public/an126.zip
Note: In the product data sheet, it is expressed as tW1L (write 1 low) minus ε (time to rise to VTH) plus tF (time to fall to VTL). The network is assumed to be a medium-distance network of standard speed, and the rise and fall times do not exceed 3 µs. Assume that the network is a small, high-speed network with rise and fall times not exceeding 0.5µs. In the data, it is expressed as tSLOT (slot time) minus the time represented by 'A'. In the data, it is expressed as tW0L (write 0 low) minus δ (time to rise to VIHMASTER) plus tF (time to fall to VTL). In the data, it is expressed as tREC (recovery time) plus δ (time to rise to VIHMASTER). In the data, it is expressed as tMSR (host sample read time) plus tF (time to fall to VTL), and then subtract 'A'. Within this range, sampling should be as late as possible in order to obtain the longest recovery time. In the data, it is expressed as tSLOT (slot time) minus 'A', and then minus 'E'. In the data sheet, it is expressed as tRSTL (time to reset to low) minus ε (time to rise to VTH) plus tF (time to fall to VTL). In the data, it is expressed as tMSP (master sample response time) plus ε (time to rise to VTH). In the data, the minimum value is expressed as tRSTL (reset low time) minus the time taken by 'I'. The 1-Wire reset operation mentioned here does not take into account the extended acknowledgment (alarm) pulse sequence used by DS2404 and DS1994. For this special case, please refer to the product data sheet. Sampling at the end of the 1-Wire reset sequence can verify that the 1-Wire bus has returned to the pull-up level. If the level is still 0, it may be a short circuit between the 1-Wire bus and ground, or the DS2404 / DS1994 issued an alarm signal. Expressed as tREC (Recovery Time) minus the time taken for 'D'. In high-speed applications, some devices require additional delay before tRSTL (low-level reset time) to ensure that the parasitic power supply of the device is fully charged. For low voltage operation, some devices may require a longer delay. Refer to the device data sheet for appropriate parameter values. Code examples The following code examples rely on two general 'C' functions outp and inp to read and write byte data from the IO port. They are usually located
// send 'databyte' to 'port' int outp (unsigned port, int databyte); // read byte from 'port' int inp (unsigned port); the constant PORTADDRESS (Figure 3) in the code is used to define the communication port address. Here we assume that bit 0 of the communication port is used to control the 1-Wire bus. Setting this bit to 1 will cause the 1-Wire bus to go low; setting this bit to 0 will release the 1-Wire bus, and the 1-Wire bus will be pulled up by the resistor or 1-Wire Pull down from the device.
The tickDelay function in the code is a user-programmed subroutine. This function is used to generate a delay of an integer multiple of 1 / 4µs. Under different platforms, the implementation of this function is also different, so it will not be described in detail here. The following is the tickDelay function declaration code, and a SetSpeed ​​function, which is used to set the delay time for standard speed and high-speed mode.
Example 1. 1-Wire timing generation // Pause for exactly 'tick' number of ticks = 0.25us void tickDelay (int tick); // Implementation is platform specific // 'tick' values ​​int A, B, C, D , E, F, G, H, I, J; // ------------------------------------ ------------------------------------------ // Set the 1-Wire timing to 'standard' (standard = 1) or 'overdrive' (standard = 0). // void SetSpeed ​​(int standard) {// Adjust tick values ​​depending on speed if (standard) {// Standard Speed ​​A = 6 * 4 ; B = 64 * 4; C = 60 * 4; D = 10 * 4; E = 9 * 4; F = 55 * 4; G = 0; H = 480 * 4; I = 70 * 4; J = 410 * 4;} else {// Overdrive Speed ​​A = 1.5 * 4; B = 7.5 * 4; C = 7.5 * 4; D = 2.5 * 4; E = 0.75 * 4; F = 7 * 4; G = 2.5 * 4; H = 70 * 4; I = 8.5 * 4; J = 40 * 4;}} The code program for basic 1-Wire operation is shown in Example 2.
Example 2. Basic 1-Wire functions // -------------------------------------------------- -------------------------------------- // Generate a 1-Wire reset, return 1 if no presence detect was found, // return 0 otherwise. // (NOTE: Does not handle alarm presence from DS2404 / DS1994) // int OWTouchReset (void) {int result; tickDelay (G); outp (PORTADDRESS, 0x00); // Drives DQ low tickDelay (H); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (I); result = inp (PORTADDRESS) & 0x01; // Sample for presence pulse from slave tickDelay (J); / / Complete the reset sequence recovery return result; // Return sample presence pulse result} // ------------------------------- ---------------------------------------------- // Send a 1-Wire write bit. Provide 10us recovery time. // void OWWriteBit (int bit) {if (bit) {// Write '1' bit outp (PORTADDRESS, 0x00); // Drives DQ low tickDelay (A); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (B); // Complete the time slot and 10us recovery} else {// Write '0' bit outp (POR TADDRESS, 0x00); // Drives DQ low tickDelay (C); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (D);}} // -------------- -------------------------------------------------- ------------- // Read a bit from the 1-Wire bus and return it. Provide 10us recovery time. // int OWReadBit (void) {int result; outp (PORTADDRESS, 0x00) ; // Drives DQ low tickDelay (A); outp (PORTADDRESS, 0x01); // Releases the bus tickDelay (E); result = inp (PORTADDRESS) & 0x01; // Sample the bit value from the slave tickDelay (F) ; // Complete the time slot and 10us recovery return result;} This program includes all bit operations on the 1-Wire bus. By calling this program, you can form a function that takes bytes as the processing object, see Example 3.
Example 3. Derived 1-Wire function // ------------------------------------------ -------------------------------------- // Write 1-Wire data byte // void OWWriteByte (int data) {int loop; // Loop to write each bit in the byte, LS-bit first for (loop = 0; loop <8; loop ++) {OWWriteBit (data & 0x01); // shift the data byte for the next bit data >> = 1;}} // ------------------------------------- ---------------------------------------- // Read 1-Wire data byte and return it // int OWReadByte (void) {int loop, result = 0; for (loop = 0; loop <8; loop ++) {// shift the result to get it ready for the next bit result >> = 1; // if result is one, then set MS bit if (OWReadBit ()) result | = 0x80;} return result;} // ----------------------- -------------------------------------------------- ---- // Write a 1-Wire data byte and return the sampled result. // int OWTouchByte (int data) {int loop, result = 0; for (loop = 0; loop <8; loop ++) {// shift the result to get it ready for the next bit result >> = 1; // If sending a '1' then read a bit else write a '0' if (data & 0x01) {if (OWReadBit ()) result | = 0x80;} else OWWriteBit (0); // shift the data byte for the next bit data >> = 1;} return result;} // ------------------------------------- ---------------------------------------- // Write a block 1-Wire data bytes and return the sampled result in the same // buffer. // void OWBlock (unsigned char * data, int data_len) {int loop; for (loop = 0; loop <data_len; loop ++) {data [loop] = OWTouchByte (data [loop]);}} // ----------------------------------------- ------------------------------------ // Set all devices on 1-Wire to overdrive speed. Return '1' if at least one // overdrive capable device is detected. // int OWOverdriveSkip (unsigned char * data, int data_len) {// set the speed to 'standard' SetSpeed ​​(1); // reset all devices if ( OWTouchReset ()) // Reset the 1-Wire bus return 0; // Return if no devices found // overdrive skip command OWWriteByte (0x3C); // set the speed to 'overdrive' SetSpeed ​​(0 ); // do a 1-Wire reset in 'overdrive' and return presence result return OWTouchReset ();} The OWTouchByte function can finish reading and writing 1-Wire bus data at the same time, and can read and write data blocks through this function. The execution efficiency is higher on some platforms, and the API provided by Maxim uses this function. Through the OWTouchByte function, the OWBlock function simplifies the sending and receiving of data blocks on the 1-Wire bus. Note: OWTouchByte (0xFF) is equivalent to OWReadByte (), and OWTouchByte (data) is equivalent to OOWriteByte (data).
These functions and the tickDelay function together form all functions necessary for 1-Wire bus to perform bit, byte and block operations. Example 4 shows an example of using these functions to read the DS2432's SHA-1 authentication page.
Example 4. Read the DS2432 example // ------------------------------------- ----------------------------------- // Read and return the page data and SHA-1 message authentication code (MAC) // from a DS2432. // int ReadPageMAC (int page, unsigned char * page_data, unsigned char * mac) {int i; unsigned short data_crc16, mac_crc16; // set the speed to 'standard' SetSpeed ​​(1) ; // select the device if (OWTouchReset ()) // Reset the 1-Wire bus return 0; // Return if no devices found OWWriteByte (0xCC); // Send Skip ROM command to select single device // read the page OWWriteByte (0xA5); // Read Authentication command OWWriteByte ((page << 5) & 0xFF); // TA1 OWWriteByte (0); // TA2 (always zero for DS2432) // read the page data for (i = 0 ; i <32; i ++) page_data [i] = OWReadByte (); OWWriteByte (0xFF); // read the CRC16 of command, address, and data data_crc16 = OWReadByte (); data_crc16 ; // delay 2ms for the device MAC computation // read the MAC for (i = 0; i <20; i ++) mac [i] = OWReadBy te (); // read CRC16 of the MAC mac_crc16 = OWReadByte (); mac_crc16 | = (OWReadByte () << 8); // check CRC16 ... return 1;} additional softwareThis application note gives 1- The basic functions of the Wire bus operation. These basic functions are the basis for building complex 1-Wire applications. An important operation ignored in this article is 1-Wire search. Through 1-Wire search, you can search for the unique ID numbers of multiple 1-Wire slave devices attached to the bus. This search method is described in detail in Application Note 187: "1-Wire Search Algorithm", and C code that implements these 1-Wire basic functions is also given.
The 1-Wire public development kit contains a lot of code written for specific devices, provided by the following links:
http: //
For more detailed information, please refer to Application Note 155: "1-Wire Software Resource Guide".
Alternative solution If it is not feasible to implement the 1-Wire master solution through software for a specific application, then as an alternative solution, a 1-Wire master chip or a synthesized 1-Wire master unit can be used.
Maxim provides 1-Wire masters written in Verilog and VHDL.
DS1WM
To obtain the Verilog / VHDL code of the 1-Wire host, please submit a technical support application.
The working method of the synthesized 1-Wire master is described in application note 120: "Communication with 1-Wire Master" and application note 119: "Embedded 1-Wire Master".
There are a variety of 1-Wire host chips that can be used as peripherals for microprocessors. The serial, 1-Wire line driver DS2480B can be easily connected to a standard serial port.
For details on the operation of the DS2480B, see Application Note 192: "Use of the DS2480B Serial Interface 1-Wire Line Driver").
This is an advanced 1-Wire line driver specially designed for long-distance 1-Wire transmission lines. In application note 244: "Excellent 1-Wire Network Driver", a more detailed 1-Wire design specifically designed for long lines is described. Wire drive.
Revision history
07/06/00: Version 1.0-the original version.
05/28/02: Version 2.0-Corrected 1-Wire reset sampling time. Added waveform diagrams, links, and more code examples.
02/02/04: Version 2.1-Added support for high-speed mode, given minimum and maximum timings, and updated code examples.
09/06/05: Version 2.2-Corrected PIO polarity in the code routine description.
Disposable Face Mask,Medical Surgical Mask , KN95 Mask,N95 Mask
Shenzhen Gmaii Technology Limited , https://www.gmaiipos.com